30 Jul 2018
A Guide to Using Social Login with Django
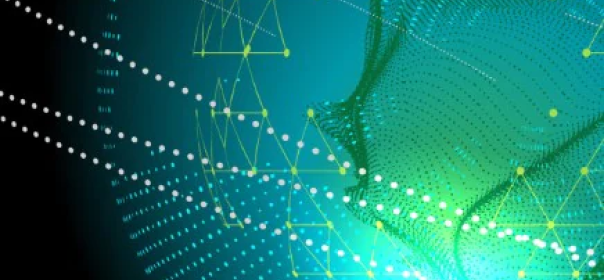

Sripathi Krishnan
#Django | 6 Min Read
In this article post, we will learn how to implement social login in a Django application by using Social auth app Django which happens to be one of the best alternatives of the deprecated library python social auth
What is Social-Auth-App-Django?
Social auth app Django is an open source python module that is available on PyPI. This is maintained under the Python Social Auth organization. Initially, they started off with a universal python module called Python social auth which could be used in any Python-based web framework. However, at the moment they have deprecated this library.
Going further, the code base was split into several modules in order to offer better support for python web frameworks. The core logic of social authentication resides in the social core module, which is then used in other modules like – social auth app django, social app flask, etc.
Installation Process
Let us now create an app called authentication. This app will contain all the views/urls related to Django social authentication.
python manage.py startapp authentication
You will have to make sure that you have created a Django project and then install social-auth-app-django from PyPI.
pip install social-auth-app-django
Then, add ‘social_django’ to your installed apps.
INSTALLED_APPS = [
...
'social_django',
'authentication',
]
Now, migrate the database:
python manage.py migrate
Now, go to http://localhost:8000/admin and you should see three models under SOCIAL_DJANGO app
Configure Authentication Backend
Now, to use social-auth-app-django’s social login, we will have to define our authentication backends in the setting.py file. Note that although we want to give our users a way to sign in through Google, we also aim to retain the normal login functionality where the username and password can be used to login to our application. In order to do that, configure the AUTHENTICATION_BACKENDS this way:
AUTHENTICATION_BACKENDS = (
'social_core.backends.google.GoogleOAuth2',
'django.contrib.auth.backends.ModelBackend',
)
Note that we have
django.constrib.auth.backends.ModelBackend
still in our AUTHENTICATION_BACKENDS. This will let us retain the usual login flow.Template and Template Context Processors
To keep it simple, we will have a plain HTML file which has a link to redirect the user for google+ login.
Go ahead and add a templates’ folder to the authentication app and create a new index.html file in it. In this html file, we will just be putting a simple anchor tag, which will redirect to social-auth-app-django’s login URL
Google+
In authentication/views.py render this template
We will now have to add context processors, which provide social auth related data to template context:
TEMPLATES = [
{
'BACKEND': 'django.template.backends.django.DjangoTemplates',
'DIRS': ['templates'],
'APP_DIRS': True,
'OPTIONS': {
'context_processors': [
'django.template.context_processors.debug',
'django.template.context_processors.request',
'django.contrib.auth.context_processors.auth',
'django.contrib.messages.context_processors.messages',
'social_django.context_processors.backends', # this
'social_django.context_processors.login_redirect', # and this
],
},
},
]
URL Configurations
So, now we have our template setup and we just need to add our index view and the Django social auth URLs in our project.
Go to the urls.py and add this code:
urlpatterns = [
url(r'^admin/', admin.site.urls),
url('^api/v1/', include('social_django.urls', namespace='social')),
url('', views.index),
]
Obtaining Google OAuth2 Credentials
To obtain the Google OAuth2 credentials, Follow these steps:
- Go to Google developer console
- You now have to create a new project. In case you do not have any (see the drop-down at the top left of your screen)
- Once the project is created you will be redirected to a dashboard. You will have to click on ENABLE API button
- Select Google+ APIs from the Social APIs section
- On the next page, click the Enable button to enable Google+ API for your project
- To generate credentials, select the Credentials tab from the left navigation menu and hit the ‘create credentials’ button
- From the drop-down, select OAuth client ID.
- On the next screen, select the application type (web application in this case) and enter your authorized javascript origins and redirect uri
For now, just set the origin as
http://localhost:8000
and the redirect URI as http://localhost:8000/complete/google-oauth2/
Hit the create button and copy the client ID and client secret
Configuration of OAuth Credentials
Now, got to setting.py and add you OAuth creadentials like this:
SOCIAL_AUTH_GOOGLE_OAUTH2_KEY =
SOCIAL_AUTH_GOOGLE_OAUTH2_SECRET =
Finally, define the LOGIN_REDIRECT_URL
LOGIN_REDIRECT_URL = '//'
For setting up credentials for other OAuth2 backend, the pattern is like this:
SOCIAL_AUTH__KEY =
SOCIAL_AUTH__SECRET =
Usage
Now that the configuration is done, we can start off using the social login in the Django application. Go to http://localhost:8000 and click the Google+ anchor tag.
It should redirect to Google OAuth screen where it asks you to sign in. Once you sign in through your Gmail account, you should be redirected to the redirect URL.
To check if a user has been created, go to the django admin
(http://localhost:8000/admin)
and click on the User social auths
table. You should see a new user there with the provider as goole-oauth2 and Uid as your gmail id.Triggering The OAuth URLs from Non-Django Templates
There are chances that you want to trigger the social login URLs from a template that is not going to pass through Django’s template context processors. For instance, when you want to implement the login in an HTML template which is developed in using ReactJS.
This is not as complicated as it looks. Just mention the href in your anchor tag like this:
Google+
The login URL for other OAuth providers is of the same pattern. If you are not sure about what the login redirect url should be, there are two ways to find the URL – either jump into their code base or try this hack –
- You can create a Django template and place an anchor tag with the respective URL as shown in the Templates and Template context processors section.
- You can remove the respective provider (in case of Google oauth2, remove
social_core.backends.google.GoogleOAuth2
from theAUTHENTICATION_BACKENDS
in settings.py - You can click the anchor tag in a Django template. Doing so, you would see the Django’s regular error template saying “Backend not found”.
- Copy the current URL in the browser and use it in your non-Django template.
Summary
We learned what Social auth app Django is and that it uses social core behind the scenes. We also learned how to implement social login in Django through templates that are processed by Django and through non-Django templates, which could be developed using JS frameworks like ReactJS.